- Typescript Daily
- Posts
- Unlocking WebAssembly's Power: Building High-Performance Modules in C++
Unlocking WebAssembly's Power: Building High-Performance Modules in C++
Learn how to build and optimize high-performance WebAssembly modules with C++! Plus, discover how you can seamlessly invoke C++ from JavaScript for blazing-fast web applications.
Welcome back to our exploration of WebAssembly (Wasm), where we dive deep into building and optimizing high-performance modules with C++. In Day 1, we discovered the incredible potential of Wasm; today, we're taking it a step further with a focus on C++, all while noting that these concepts can be applied to other languages like C, TypeScript, Rust, and more.
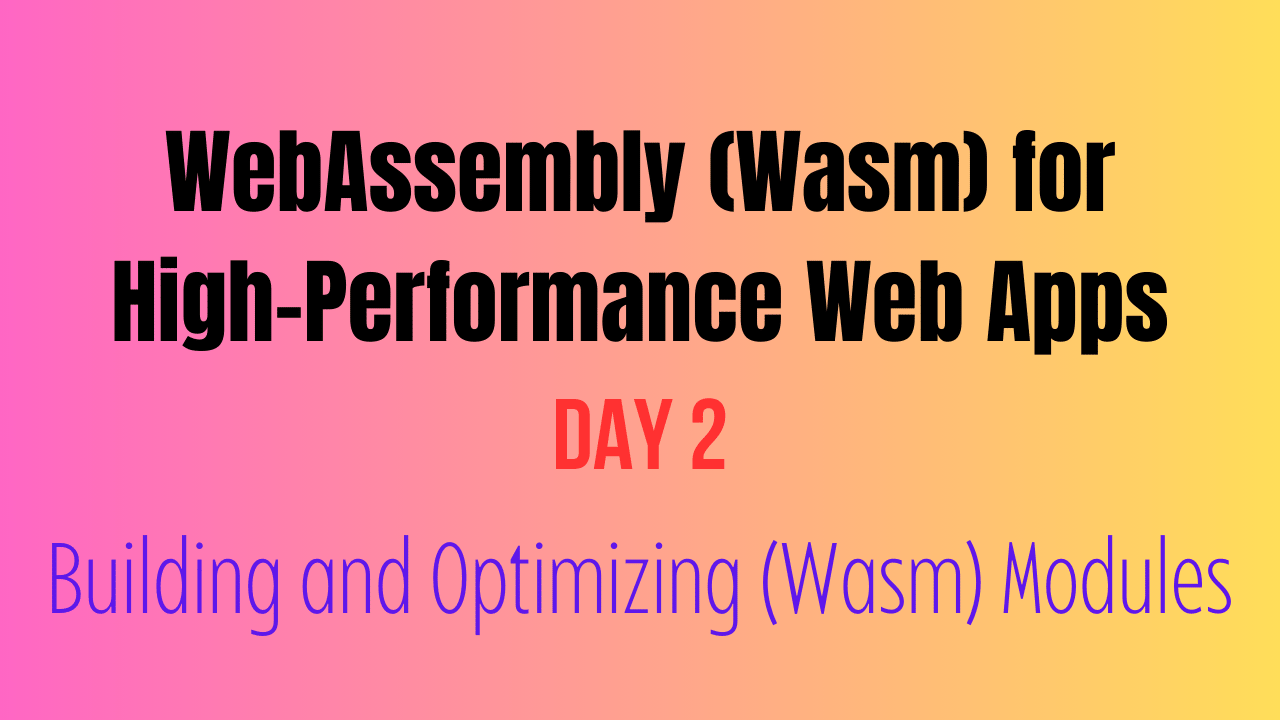
The Challenge: Crafting Efficient WebAssembly Modules
Creating efficient WebAssembly modules is essential for delivering high-performance web applications. Whether you're using C++, C, TypeScript, Rust, or other languages, optimizing your code can make a world of difference. Let's explore this with real-world examples.
C++ and WebAssembly: A Dynamic Duo
C++ is renowned for its performance and control, making it an ideal choice for writing code that compiles to WebAssembly. It provides low-level control over memory and CPU operations, enabling developers to optimize code for speed and efficiency. However, keep in mind that these concepts can be applied to other languages as well.
Getting Started: Setting Up Your Environment
Before we dive into coding, ensure your development environment is ready:
Emscripten: Install Emscripten, a toolchain for compiling C/C++ code to WebAssembly. You can find it here.
Node.js: Install Node.js to run JavaScript/TypeScript and work with WebAssembly outside of the browser.
A Code Editor: Any code editor of your choice, such as Visual Studio Code.
Creating a C++ Module
Let's start by creating a C++ module that performs a real-world task. In this example, we'll build a module that calculates the nth Fibonacci number using an iterative approach. Here's the C++ code (fibonacci.cpp):

This C++ module calculates the nth Fibonacci number using an iterative approach.
Compiling C++ to WebAssembly with Emscripten
To compile this C++ module to WebAssembly, we'll use Emscripten. Compile the C++ code to WebAssembly:

This generates a fibonacci.js
file that can be used to interact with the WebAssembly module from JavaScript.
Consuming WebAssembly in JavaScript
Now, let's see how you can consume this WebAssembly module in JavaScript. Create an HTML file (index.html) and JavaScript file (app.js) as follows:


Here, we import the WebAssembly module and call the fibonacci
function to calculate the Fibonacci of 5, printing the result to the console.
Optimizing WebAssembly Modules
Optimizing WebAssembly modules, whether written in C++, C, TypeScript, Rust, or other languages, follows similar principles:
Minimize Memory Usage: Efficient memory usage is crucial. Avoid excessive memory allocations.
Optimize Loops: In performance-critical loops, reduce unnecessary iterations and use efficient algorithms.
Leverage Profiling: Use profiling tools to identify bottlenecks and optimize accordingly.
Real-World Scenario: Real-Time Image Processing
Imagine you're developing a web application that applies real-time filters to images, enhancing user experiences dramatically. By optimizing your image processing algorithms in WebAssembly, you can achieve lightning-fast image transformations. These concepts apply not only to C++ but also to other languages, enabling you to tackle complex tasks with speed and efficiency.
Invoking C++ from JavaScript at Runtime
One of the key strengths of WebAssembly is the ability to invoke C++ functions from JavaScript at runtime. In our example, we imported the WebAssembly module and called the fibonacci
function as if it were a regular JavaScript function. This seamless integration between languages empowers you to leverage the performance of C++ while enjoying the flexibility of JavaScript.
What's Next?
In Day 3, we'll dive even deeper into integrating WebAssembly with JavaScript, allowing you to create dynamic web experiences that seamlessly combine the strengths of both technologies. Stay tuned for more exciting insights and real-world examples in our Wasm series!
In the meantime, keep experimenting with C++, TypeScript, Rust, or your language of choice in the WebAssembly world. Get ready to revolutionize your web development projects!
Reply